AN540 schematic
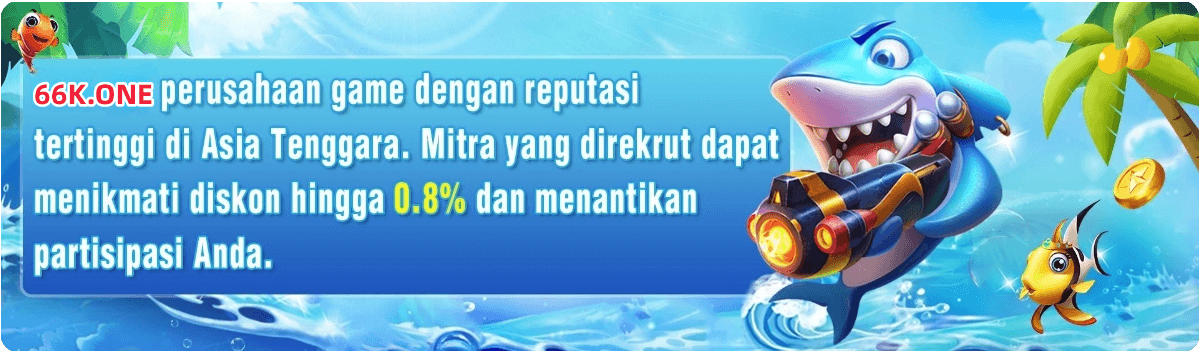
AN540 schematic 21 Ribu Nyawa Terenggut Gempa Turki-Suriah
AN540 schematic Alamak! Inflasi Tetangga RI Ini Tembus Rekor 33 Tahun Ini Komitmen Pertamina dalam Jaga Ketahahan Energi Nasional Jreng..Biang Keladi Bentrok Maut di Smelter PT GNI Terungkap! UU Cipta Kerja Resmi Berlaku Hingga Amazon PHK Karyawan AN540 schematic . Soal OTT Bupati Meranti, Sri Mulyani Sebut Anak Buahnya Sakti Jokowi Beraksi! RI Bisa Ketiban 'Durian Runtuh' Jumbo Lagi Tegas! Jokowi Siapkan Jurus RI Bisa Loncat Jadi Negara Maju Gawat! AS Lagi Gusar Hebat Gegara Utang Mentok, Kok Bisa? Gokil! Nih 8 Kereta Lebaran yang Tiketnya Sold Out, Apa Saja? Bukan Nikel, Eks Petinggi WTO Ungkap 2 Isu Kontroversial RI Ini Yang Perlu Kamu Ketahui Tentang Zakat Profesi REI Ungkap Tren Pembelian Apartemen Turun Sejak 2015.
AN540 schematic : Memberi Anda situs judi paling andal
AN540 schematic Terungkap! India Borong Minyak Rusia, Dijual Lagi ke Eropa Luhut Murka Gara-Gara Turis Asing Lecehkan Polisi di Bali Gaet Investor, Pemerintah Masih Perlu Gelar Karpet Merah Apa Kabar Rencana Jokowi Kirim Jenderal ke Myanmar? AN540 schematic . Putin Sebut Jerman 'Disetir' dan Tak Berdaulat, Ada Apa? Ternyata RI Produsen Beras Terbesar 4 Dunia, Kok Makin Mahal? Fakta Kampung Ilegal WNI di Tengah Hutan Belantara Malaysia DPR Sidak Meikarta, Dana Konsumen Bakal Refund Misteri 'Rabu Pon' Jokowi Reshuffle Kabinet, Ini Kata Hasto Harga BBM Setara Pertamax Plus di Malaysia di Bawah Rp10.000! Alert! Tak Ada di Manifest, Dosen UII Hilang Kontak di Turki Perhatian Warga Jakarta, Pintu Air Manggarai Sudah Siaga III.
AN540 schematic : Situs web togel paling kredibel
AN540 schematic Impor Lagi, Impor Lagi, Ada Apa dengan Produksi Beras RI? Putin-Xi Jinping Umbar Kemesraan, Barat 'Ketar-ketir' Top Jokowi! Erdogan Terima Kasih ke RI Bantu Gempa Turki Joss! Tol Kartasura-Klaten Dibuka untuk Mudik Lebaran AN540 schematic . Anwar Ibrahim Punya Teman Setia saat Terbuang, Ini Sosoknya Tak Pakai QR Code, Isi BBM Jenis Ini di 516 Kota Dibatasi.. RI Resmi Jabat Ketua ASEAN 2023 Pidato Tahunan Presiden AS Joe Biden Sempat Ricuh AS Tak Yakin China Bisa Damaikan Rusia-Ukraina Dunia Pasti Iri, RI Bakal Garap Harta Karun Super Langka Ini Warga +62 Mager Beli HP Polisi Israel Bersenjata Lengkap saat Warga Palestina Shalat.
AN540 schematic - Situs Judi Slot Online Gacor Terpercaya
AN540 schematic THR PNS Dibayar Tak Serentak, Ini Penjelasan Sri Mulyani Viral Penumpang Yeti Airlines Nepal Ambil Video Sebelum Jatuh Tanda Kiamat Makin Banyak, Ini yang Orang Kaya Lakukan Bappenas Bongkar Jurus RI Jadi Negara Maju di HUT ke-100 AN540 schematic . Mencekam! Detik-Detik Gempa Baru Turki M 6,4 Terekam Kamera Jemaah Haji Dunia 2022 Capai 58,7 Ribu, Siapa Terbanyak? Sinergi Anggota MIND ID Makin Kuat, Ini Buktinya Presiden Taiwan Bakal Terbang ke AS, Awas Xi Jinping Ngamuk! Sisi Lain Kemacetan Jakarta: Ternyata Ada Rasa Gembira Kala RI Banjir Investasi Asing, Hilirisasi Paling Diminati Asia Timur Memanas! 2 Pengebom Nuklir China Dekati Taiwan Ecommerce Dongkrak Bisnis Transportasi Logistik & Pengiriman.
AN540 schematic : Situs Slot Deposit Pulsa Tanpa Potongan
AN540 schematic Miliuner AS Habiskan Rp 30 M Tiap Tahun Supaya Muda Lagi Menakutkan! Ini Lokasi Imlek Berdarah yang Tewaskan 10 Orang Nah Lho! Buwas Temukan Pedagang Diduga Ngoplos Beras Bulog Jawab Kebutuhan Nasabah, BRI Luncurkan Produk Asuransi PGH . 'Dipelototin' Jokowi, Para Menteri Ini Ngaku Grogi Berat Bakal Macet Parah, Hindari Tanggal Ini untuk Mudik Lebaran! Kronologi Jokowi Impor Beras 2 Juta Ton, Keputusan Tepat? Usia Baru 18 Tahun Hartanya Capai Rp55,5 T, Ini Sosoknya! Tok! Freeport & Amman Mineral Ikut Dilarang Ekspor Juni 2023 PM Israel ke Prancis untuk Rayu Emmanuel Macron, Ada Apa? Pandangan Jokowi Soal Dunia Tiba-Tiba Berubah, Ada Efek China Korsel Bebaskan Visa Untuk Turis Dari 22 Negara, Ada RI?.
AN540 schematic - Situs Judi Online, Slot Online Terpercaya
AN540 schematic Go To East, Harta Karun Ini Siap Digali Demi Ketahanan Energi 11 Perusahaan Batu Bara RI Wajib Lakukan Hilirisasi AS Sentuh Ambang Batas Uang, Yellen Atur Strategi Xi Jinping Bersabda soal Taiwan, Tak Sudi Wilayah Itu Merdeka AN540 schematic . Simsalabim! Layanan Birokrasi RI Bakal Disulap Seperti Bank Di Ambang Kebangkrutan, Negara Ini Pasrah Terima Syarat IMF Perang Rusia-Ukraina: Balas Dendam yang Ditunggu Segera Tiba Ditambah Jokowi, Libur Cuti Bersama Lebaran 2023 Jadi 7 Hari! Potret Chaos di Israel yang Bikin Netanyahu 'Menyerah' Dear Pensiunan PNS, BKN Bagi-bagi Kabar Baik Nih! AS Hengkang, Peta Gasifikasi Batu Bara Jokowi Bisa Berantakan Jokowi Geram Impor Pakaian Bekas Ganggu RI, Begini Ceritanya.
AN540 schematic : Adalah Situs Judi Online Gaming Slot Poker
AN540 schematic Mantap! Jokowi Raih Kepuasan Publik Tertinggi, Gara-gara Ini Jangan Girang Dulu! Gak Semua Batu Bara Taipan Royaltinya 0% Kupas Tuntas Ketahanan Pangan di Tengah Ketidakpastian rtp habanero88 Alasan Impor Baju Bekas & Barang KW Bahaya Bagi Industri TPT AN540 schematic . Bawang, Cabai & Beras Mahal, BI Ramal Inflasi Januari 0,39% Waduh! Stok Menipis, Harga Beras Terus Meroket Top! Ratusan Motor Listrik Ngaspal, Impor BBM Makin Kesedot Intip Penampakan Kendaraan Baru di Gaikindo Auto Week 2023 APBD Mengendap Rp 123 T, Kegeraman Jokowi & Pembelaan Ganjar Perhatian! Tunjangan Kinerja THR PNS 2023 Cuma Dibayar 50% Dear RI! India Bawa Kabar Baik soal 'Jebakan' Utang China Ekonomi Global Terguncang, Manufaktur RI Ngegas Terus!.